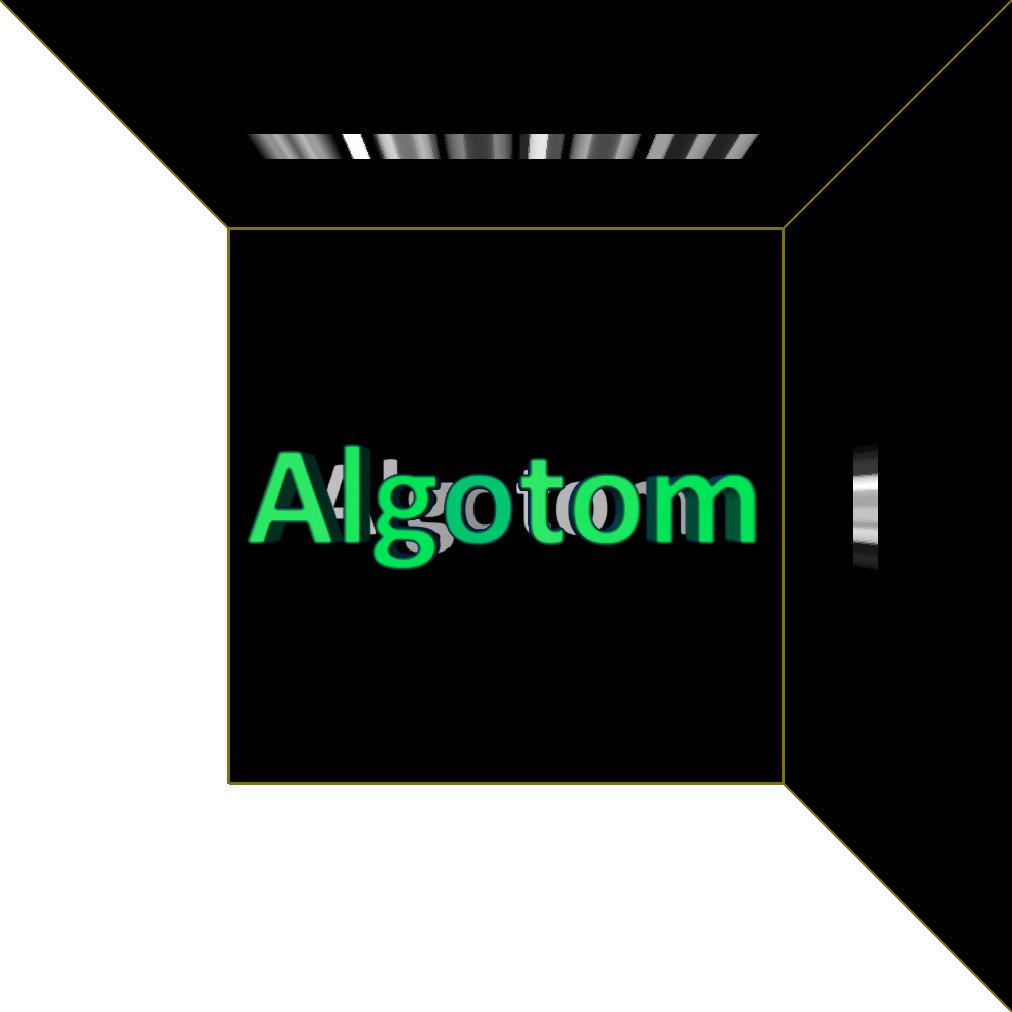
Data processing algorithms for tomography¶
Welcome to Algotom’s documentation about data processing algorithms for tomography. This documentation is not only to explain functions available in the Algotom package but also to present tomography-related tutorials, technical notes, and applications.
Source code: https://github.com/algotom/algotom
Table of Contents¶
- 1. Basic tutorials
- 1.1. Python for tomography scientists as beginners
- 1.2. Common data format at synchrotron facilities
- 1.3. Basic components of an X-ray tomography system
- 1.4. Basic workflow for processing tomographic data
- 1.5. Parallel processing in Python
- 1.6. Alignment for a parallel-beam tomography system
- 2. Features
- 3. Installation
- 4. Demonstrations
- 4.1. Setting up a Python workspace
- 4.2. Exploring raw data and making use of the input-output module
- 4.3. Methods and tools for removing ring artifacts
- 4.3.1. Improvements
- 4.3.2. Tools for designing ring removal methods
- 4.3.2.1. Back-and-forth sorting
- 4.3.2.2. Separation of frequency components
- 4.3.2.3. Polynomial fitting along an axis
- 4.3.2.4. Wavelet decomposition and reconstruction
- 4.3.2.5. Stripe interpolation
- 4.3.2.6. Transformation between Cartesian and polar coordinate system
- 4.3.2.7. Transformation between sinogram space and reconstruction space
- 4.4. Comparison of ring removal methods on challenging sinograms
- 4.5. Complete workflow for processing tomographic data
- 4.5.1. Assessing raw data
- 4.5.2. Reconstructing several slices
- 4.5.3. Finding the center of rotation
- 4.5.4. Tweaking parameters of preprocessing methods
- 4.5.5. Choosing a reconstruction method
- 4.5.6. Performing full reconstruction
- 4.5.7. Automating the workflow
- 4.5.8. Downsampling, rescaling, and reslicing reconstructed volume
- 4.5.9. Common mistakes and useful tips
- 4.5.10. Data analysis
- 5. Technical notes
- 6. Update notes
- 7. API Reference
- 7.1. Input-output
- 7.1.1.
algotom.io.converter
- 7.1.2.
algotom.io.loadersaver
load_image()
get_hdf_information()
find_hdf_key()
load_hdf()
make_folder()
make_file_name()
make_folder_name()
find_file()
save_image()
open_hdf_stream()
load_distortion_coefficient()
save_distortion_coefficient()
get_hdf_tree()
get_reference_sample_stacks_dls()
get_reference_sample_stacks()
get_tif_stack()
get_image_stack()
load_image_multiple()
save_image_multiple()
- 7.1.1.
- 7.2. Pre-processing
- 7.2.1.
algotom.prep.calculation
make_inverse_double_wedge_mask()
calculate_center_metric()
coarse_search_cor()
fine_search_cor()
downsample_cor()
find_center_vo()
calculate_curvature()
correlation_metric()
search_overlap()
find_overlap()
find_overlap_multiple()
find_center_360()
complex_gradient()
find_shift_based_phase_correlation()
find_center_based_phase_correlation()
find_center_projection()
calculate_reconstructable_height()
calculate_maximum_index()
- 7.2.2.
algotom.prep.conversion
- 7.2.3.
algotom.prep.correction
- 7.2.4.
algotom.prep.filtering
- 7.2.5.
algotom.prep.removal
remove_stripe_based_sorting()
remove_stripe_based_filtering()
remove_stripe_based_fitting()
remove_large_stripe()
remove_dead_stripe()
remove_all_stripe()
remove_stripe_based_2d_filtering_sorting()
remove_stripe_based_normalization()
remove_stripe_based_regularization()
remove_stripe_based_fft()
remove_stripe_based_wavelet_fft()
remove_stripe_based_interpolation()
check_zinger_size()
select_zinger()
remove_zinger()
generate_blob_mask()
remove_blob_1d()
remove_blob()
- 7.2.6.
algotom.prep.phase
get_quality_map()
get_weight_mask()
unwrap_phase_based_cosine_transform()
unwrap_phase_based_fft()
unwrap_phase_iterative_fft()
reconstruct_surface_from_gradient_FC_method()
reconstruct_surface_from_gradient_SCS_method()
find_shift_between_image_stacks()
find_shift_between_sample_images()
align_image_stacks()
get_transmission_dark_field_signal()
retrieve_phase_based_speckle_tracking()
- 7.2.1.
- 7.3. Reconstruction
- 7.3.1.
algotom.rec.reconstruction
make_smoothing_window()
make_2d_ramp_window()
apply_ramp_filter()
back_projection_gpu()
back_projection_gpu_chunk()
back_projection_cpu()
fbp_reconstruction()
make_circular_ramp_window()
apply_circular_ramp_filter()
bpf_reconstruction()
generate_mapping_coordinate()
dfi_reconstruction()
gridrec_reconstruction()
astra_reconstruction()
find_center_based_slice_metric()
find_center_visual_slices()
- 7.3.2.
algotom.rec.vertrec
vertical_back_projection_cpu()
vertical_back_projection_cpu_chunk()
vertical_back_projection_gpu()
vertical_back_projection_gpu_chunk()
vertical_reconstruction()
vertical_reconstruction_multiple()
vertical_reconstruction_different_angles()
find_center_vertical_slice()
find_center_visual_vertical_slices()
- 7.3.1.
- 7.4. Post-processing
- 7.5. Utilities
- 7.5.1.
algotom.util.calibration
normalize_background()
normalize_background_based_fft()
invert_dot_contrast()
calculate_threshold()
binarize_image()
get_dot_size()
check_dot_size()
select_dot_based_size()
calculate_distance()
fit_points_to_ellipse()
find_tilt_roll_based_linear_fit()
find_tilt_roll_based_ellipse_fit()
find_tilt_roll()
- 7.5.2.
algotom.util.simulation
- 7.5.3.
algotom.util.utility
parallel_process_slices()
apply_method_to_multiple_sinograms()
mapping()
make_circle_mask()
sort_forward()
sort_backward()
separate_frequency_component()
generate_fitted_image()
detect_stripe()
calculate_regularization_coefficient()
make_2d_butterworth_window()
make_2d_damping_window()
apply_wavelet_decomposition()
apply_wavelet_reconstruction()
check_level()
apply_filter_to_wavelet_component()
interpolate_inside_stripe()
rectangular_from_polar()
polar_from_rectangular()
transform_slice_forward()
transform_slice_backward()
make_2d_gaussian_window()
apply_gaussian_filter()
apply_1d_regularizer()
apply_regularization_filter()
transform_1d_window_to_2d()
detect_sample()
fix_non_sample_areas()
locate_slice()
locate_slice_chunk()
generate_spiral_positions()
find_center_visual_sinograms()
find_center_visual_slices()
- 7.5.4.
algotom.util.correlation
- 7.5.1.
- 7.1. Input-output
- 8. Credits
- 9. Highlights
- 10. Quick links